- https://isc.sans.edu/diary/Introduction+to+analysing+Go+binaries/24770
- https://docs.google.com/document/d/1lyPIbmsYbXnpNj57a261hgOYVpNRcgydurVQIyZOz_o/pub
- https://github.com/dor2727/golang_function_rename
- https://tuanlinh.gitbook.io/ctf/golang-function-name-obfuscation-how-to-fool-analysis-tools
- https://gitlab.com/zaytsevgu/goutils/blob/master/go_renamer.py
- https://harpaz.wordpress.com/category/golang/golang-internals/
Category Archives: Uncategorized
Mandelbrot Scratch
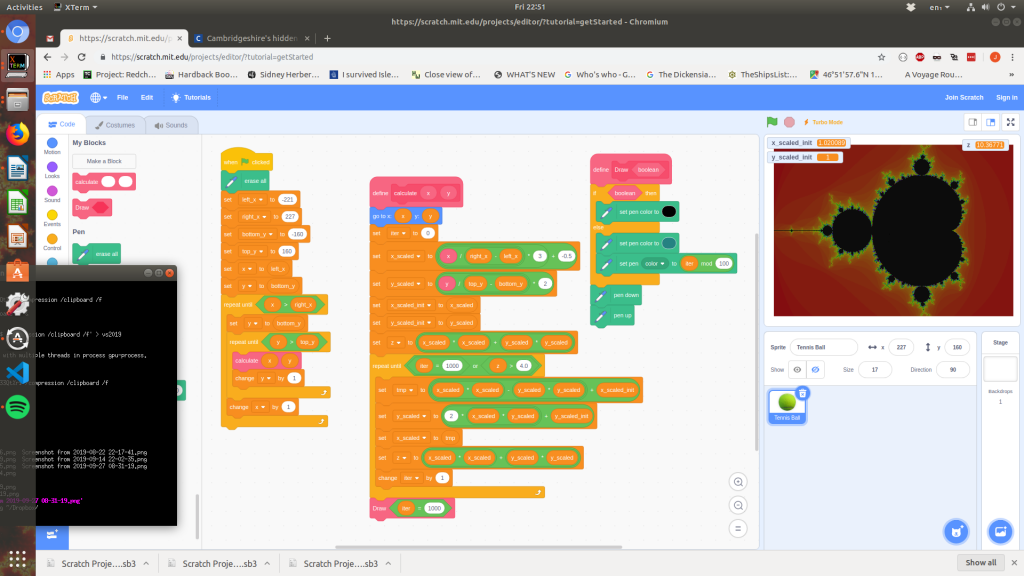
By the magic of the Pen extension, Scratch can plot coloured pixels. “Turbo Mode” is also a help here, for speed. Oddly you can set the colour (0-100) with a single command, but not the saturation and brightness, so you have to set the pen to a phoney colour if it was black before.
Of course (?) you need a fake sprite to attach the program to, in this case it’s an invisible tennis ball.
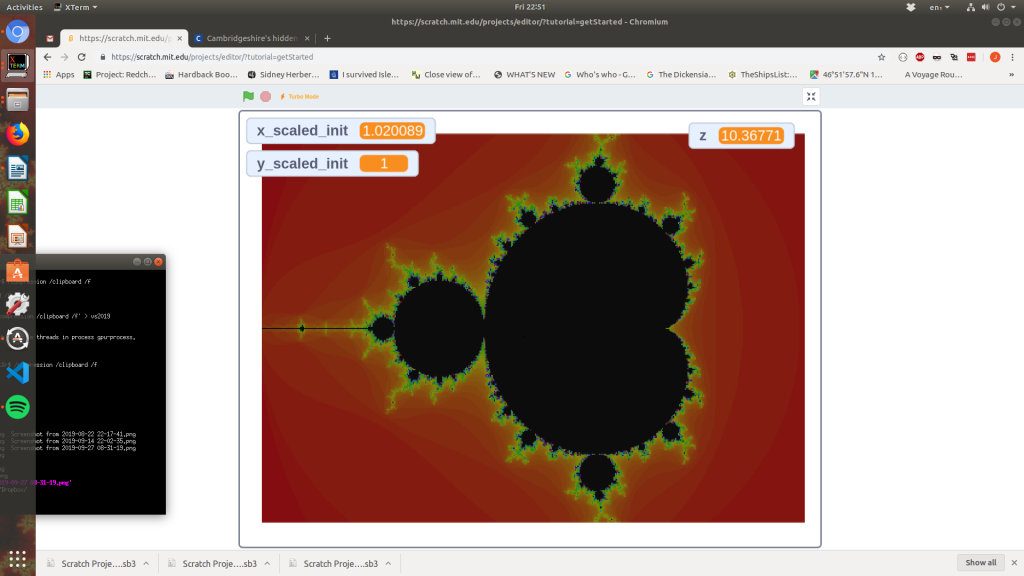
Scaling the screen to the Mandelbrot domain – x in [-1, 2.5], y in [-1,1] – is a bit trial and error when working out how big the screen is pixels, but the result is good enough.
HOWTO: grpc-gateway server in Go
Imagine we have a simple protobuf definition, below. It defines a message called Fen
which contains a single string field, fen
, and an RPC call, Position
, which takes no parameters and returns a Fen
message.
(FEN is Forsyth-Edwards Notation, a compact text format for describing the state of a game of chess)
syntax = "proto3"; package chess; import "google/protobuf/empty.proto"; import "google/api/annotations.proto"; service Chess { rpc Position (google.protobuf.Empty) returns (Fen) { option (google.api.http) = { get: "/position" }; }; }; message Fen { string fen = 1; };
We want to make a REST server implementing the interface defined by the protocol buffer. Using the namely/gen-grpc-gateway
container, this is relatively simple to do. First we generate the protobufs code for the Go language (the ‘-l go
‘ switch) which we’ll need for the grpc server (or clients). Then we generate the REST gateway itself.
#!/bin/bash GOPATH=/home/xxx/yyy export GOPATH PROTOC_CONTAINER=namely/protoc-all:1.11 GRPC_GATEWAY=namely/gen-grpc-gateway:latest ME=$(id -u):$(id -g) docker pull $PROTOC_CONTAINER docker run \ --user $ME \ --rm \ -v $PWD/proto:/defs \ $PROTOC_CONTAINER -d /defs -l go docker run \ -v $PWD/proto:/defs \ --user $ME \ $GRPC_GATEWAY -f /defs/chess.proto -s Chess docker build -t my-grpc-gateway proto/gen/grpc-gateway cd src/server go get && go install go build .
This done, we can implement the grpc server using the generated code, which we import:
package main import ( "context" "fmt" "log" "net" "server/pb-go" // Generated code "github.com/golang/protobuf/ptypes/empty" "google.golang.org/grpc" ) type chessServer struct {} func (c *chessServer) Position(ctx context.Context, empty *empty.Empty) (*chess.Fen, error) { return &chess.Fen{Fen: "zzzzz"}, nil } func main() { lis, err := net.Listen("tcp", fmt.Sprintf(":%d", 8080)) if err != nil { log.Fatalf("failed to listen: %v", err) } log.Print("Listening on port 8080 (grpc)") grpcServer := grpc.NewServer() chess.RegisterChessServer(grpcServer, &chessServer{}) grpcServer.Serve(lis) }
Then, the system is completed by launching the grpc REST gateway and the grpc server at the same time. Usually this would be done via docker-compose to wrap them both up nicely, but I haven’t bothered here.
#!/bin/bash docker stop grpcgate docker run \ --rm \ --name "grpcgate" \ -d \ -p 9000:80 \ my-grpc-gateway --backend=192.168.1.8:8080 echo "GRPC gateway on localhost:9000" ./src/server/server